Declaring unnamed arguments in SwiftUI
10 Oct 2020
Swift has long had the ability to omit argument labels to aid readability:
// With labelled arguments.
func greet(name: String) {
print("Hello \(name)!")
}
greet(name: "Vanessa Vanjie Matteo")
// Without labelled arguments, much nicer.
func greet(_ name: String) {
print("Hello \(name)!")
}
greet("Vanessa Vanjie Matteo")
I wanted to do this in a SwiftUI view, but I couldn’t figure out how. Here’s my original view:
struct Heading: View {
let title: String
var body: some View {
HStack {
Text(title).font(.title3).fontWeight(.semibold)
Spacer()
}
}
}
// I have to set the 'title' argument every time I call it:
Heading(title: "Section Heading")
My colleagues Zsolt and Hugo showed me how to do this. SwiftUI views have an init
method that is generated behind the scenes. I can just declare my own:
struct Heading: View {
let title: String
init(_ title: String) {
self.title = title
}
var body: some View {
HStack {
Text(title).font(.title3).fontWeight(.semibold)
Spacer()
}
}
}
// Now I can call it in a nicer way!
Heading("Section Heading")
In hindsight this seems really obvious, but I couldn’t figure it out for ages!
Thanks for reading.
To get in touch, email me or find me on Mastodon or Twitter.
If you liked this post, you'll love my iOS apps. Check them out below.
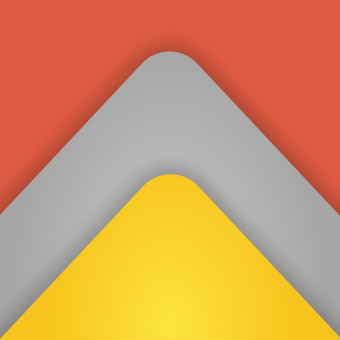
Personal Best
Level up your workouts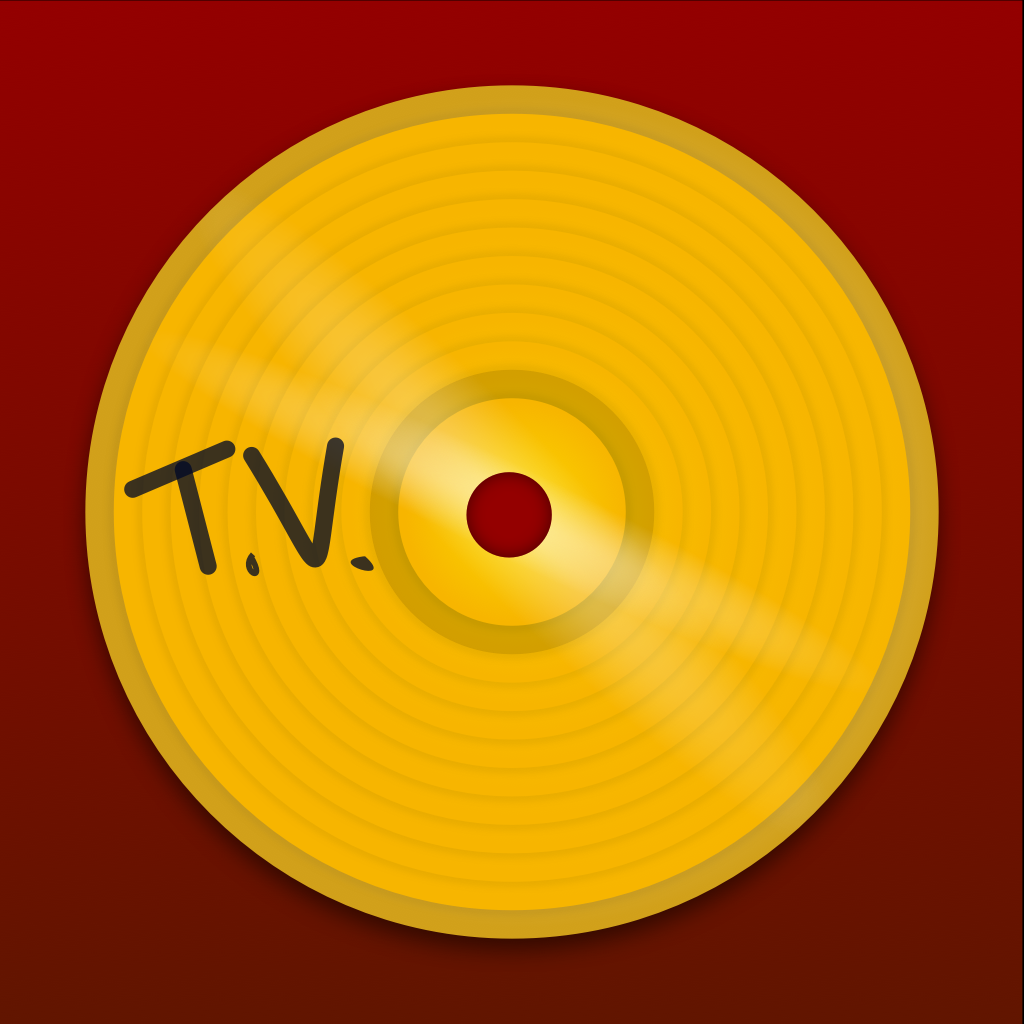
Taylor's Version
Upgrade Taylor Swift songs in your playlists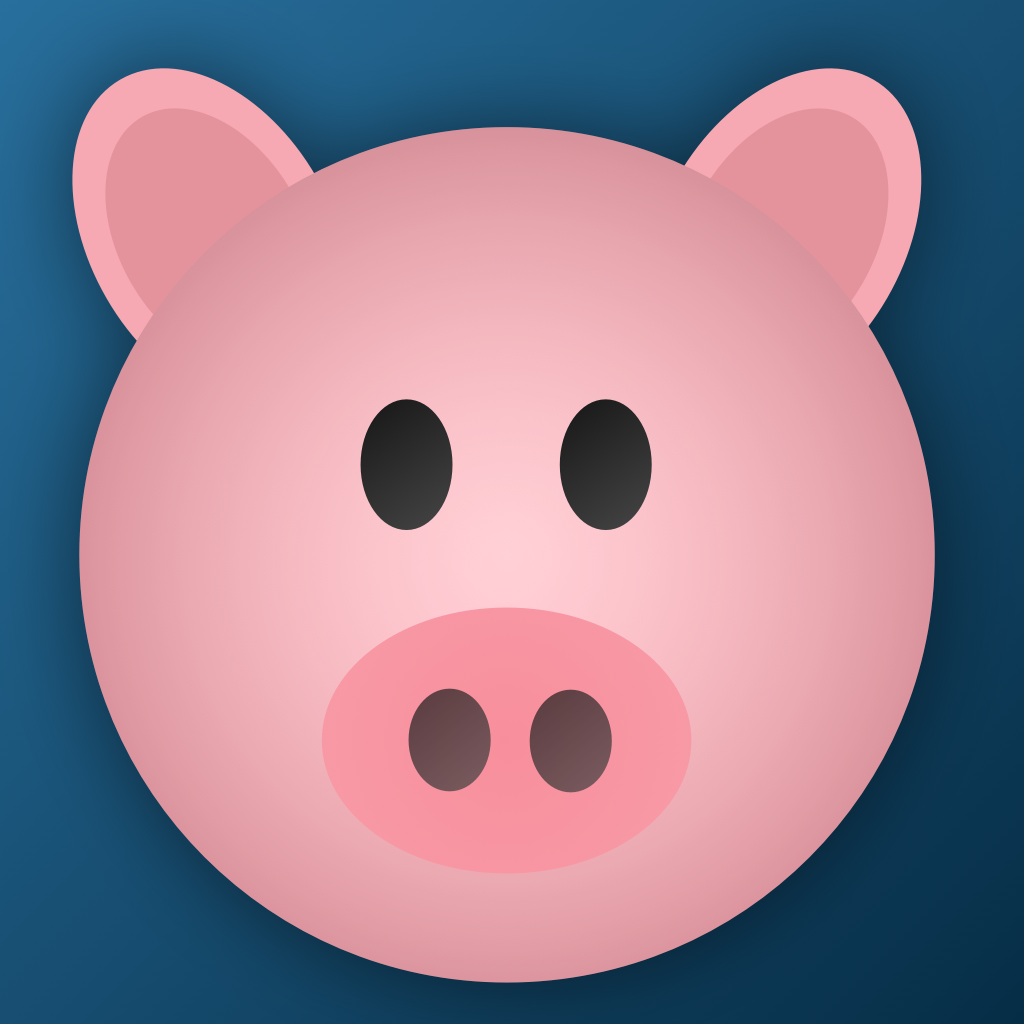
SalaryPig
Meet Trevor, the salary-tracking pig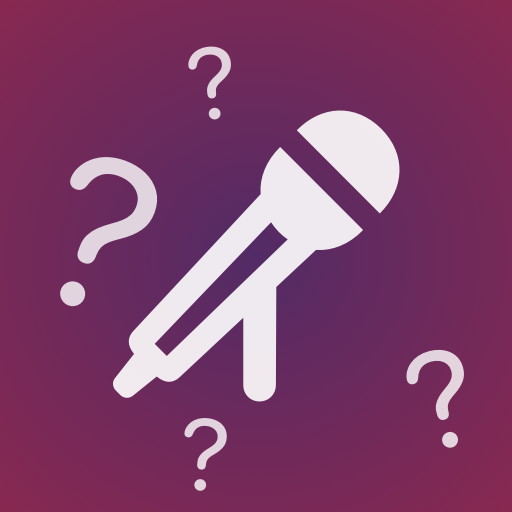